Unlocking Python's Special Methods: A Deep Dive
Written on
Chapter 1: Introduction to Python's Special Methods
Python is a multifaceted programming language that grants developers significant flexibility and power. A standout feature of Python is its support for object-oriented programming (OOP), enabling the creation of custom classes and objects that encapsulate both data and functionality. A crucial aspect of this is Python's collection of special methods, often referred to as dunder methods. These methods are defined within class definitions to facilitate specific behaviors and are characterized by double underscores surrounding their names, such as __init__ and __str__.
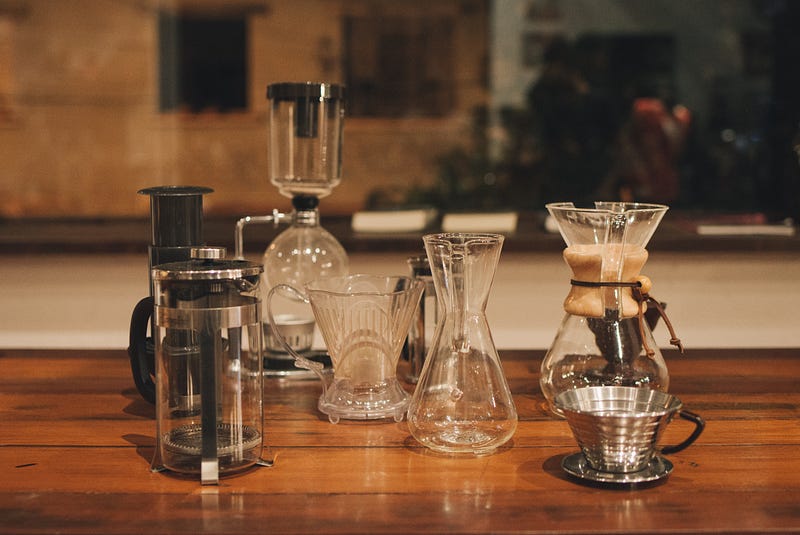
Photo by Nathalia Segato on Unsplash
In this guide, we will examine Python's special methods in detail, including their syntax and practical applications. Gaining a solid understanding of these methods will empower you to write more expressive and versatile code, fully leveraging Python's object-oriented functionalities.
Chapter 2: Overview of Special Methods
Let's briefly outline the various special methods available in Python:
- `__init__(self[, args...])`: This method is invoked when an instance of a class is instantiated, initializing its attributes based on the provided arguments.
- `__str__(self)`: This method generates a string representation of a class instance, making it useful for debugging and print statements.
- `__repr__(self)`: Similar to __str__, this method returns a string representation that aims to recreate the instance and is also beneficial for debugging.
- `__len__(self)`: This method returns the number of items in an object, commonly used with sequences like lists and strings.
- `__getitem__(self, key)`: This method is triggered when an object is accessed via indexing (e.g., obj[0]), returning the corresponding value.
- `__setitem__(self, key, value)`: This method is called when a value is assigned to an object using indexing (e.g., obj[0] = 42), setting the designated item's value.
- `__delitem__(self, key)`: This method is called when an item is removed from an object via indexing (e.g., del obj[0]), deleting the specified item.
- `__iter__(self)`: This method returns an iterator for the object, allowing iteration over its elements.
- `__next__(self)`: This method provides the next value from the iterator and is used in conjunction with the iter method.
- `__contains__(self, item)`: This method checks for the presence of an item within the object, returning True or False.
- `__add__(self, other)`: This method enables the addition of two objects, invoked with the + operator.
- `__sub__(self, other)`: This method allows for the subtraction of one object from another, used with the - operator.
- `__mul__(self, other)`: This method facilitates multiplication of two objects, invoked using the * operator.
- `__truediv__(self, other)`: This method returns the result of dividing one object by another, called with the / operator.
- `__eq__(self, other)`: This method checks for equality between two objects, returning True or False, typically invoked with the == operator.
- `__ne__(self, other)`: This method checks if two objects are not equal, returning True or False, and is used with the != operator.
- `__lt__(self, other)`: This method determines if one object is less than another, returning True or False, and is called using the < operator.
- `__gt__(self, other)`: This method checks if one object is greater than another, returning True or False, typically invoked with the > operator.
- `__le__(self, other)`: This method checks if one object is less than or equal to another, returning True or False, called with the <= operator.
- `__ge__(self, other)`: This method evaluates if one object is greater than or equal to another, returning True or False, and is invoked with the >= operator.
- `__bool__(self)`: This method returns the boolean value of an object, commonly utilized in conditional statements.
- `__hash__(self)`: This method generates a hash value for an object, essential in hash-based data structures like dictionaries and sets.
- `__call__(self, args, kwargs)`: This method allows the object to be callable like a function, accepting arguments and keyword arguments.
- `__getattr__(self, name)`: This method is triggered when an attribute is accessed but not found in the object, enabling dynamic attribute generation.
- `__setattr__(self, name, value)`: This method is called when an attribute is assigned to an object, useful for validation or modification of attribute values.
- `__delattr__(self, name)`: This method is invoked when an attribute is removed from an object, often used for cleanup tasks.
- `__dir__(self)`: This method returns a list of all attribute and method names of an object.
- `__format__(self, format_spec)`: This method produces a formatted string representation of an object.
- `__enter__(self)`: This method is called when entering a with statement, useful for setup tasks.
- `__exit__(self, exc_type, exc_value, traceback)`: This method is called upon exiting a with statement, allowing for cleanup tasks.
Understanding these special methods is vital for anyone looking to harness Python's object-oriented capabilities. By effectively implementing them within your custom classes, you can produce powerful and expressive code that maximizes Python's features. Whether you are just starting out or are an experienced Python programmer, mastering these methods is essential for crafting high-quality code.
The first video titled "The Complete Guide to Python" offers a thorough overview of Python programming, emphasizing the importance of special methods within OOP.
The second video, "Python Complete Course #32: Python Special Methods," provides an in-depth look at special methods, guiding viewers through practical examples and applications.