Mastering Pointers in Go: A Comprehensive Guide
Written on
Chapter 1: Introduction to Pointers
In the Go programming language, a pointer serves as a unique type that allows you to refer to a specific value. Gaining a deeper understanding of pointers can enhance your programming capabilities in Go.
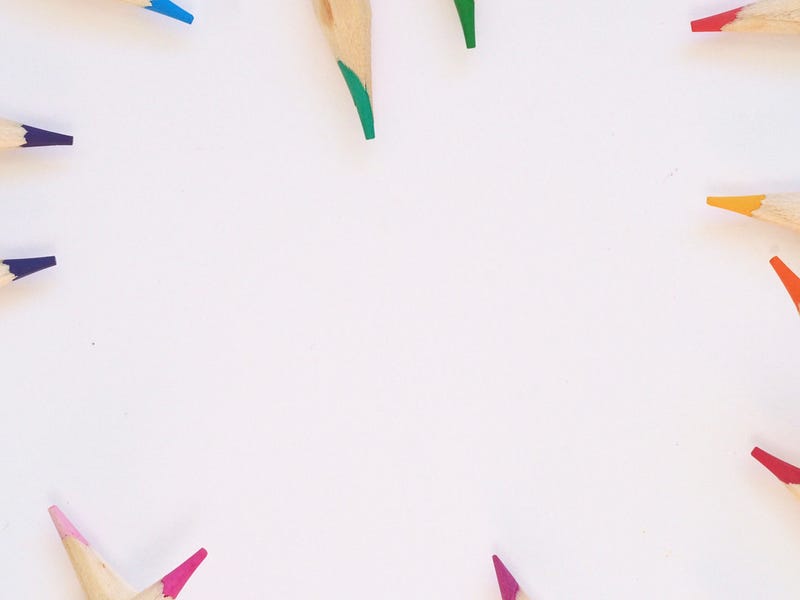
Photo by Jess Bailey on Unsplash
This article aims to clarify pointers and their usage, providing insights that I hope will be beneficial.
Section 1.1: Understanding Memory and Variables
Think of computer memory as a series of sequential boxes arranged in a line. Each box is identified by a distinct number that increases incrementally. This unique identification number is known as the memory address.

A variable serves as a simple, alphanumeric label for a memory location allocated by the compiler. When you declare a variable, you receive a memory address from the pool of available memory.
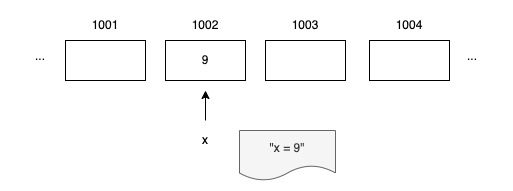
Section 1.2: What Are Pointers?
A pointer is a value that represents the address of a variable. Essentially, it indicates where a value is stored in memory. By using a pointer, you can access or modify the value of a variable indirectly, without needing to know the variable's name, if it even has one.
For instance, in the following example, the expression &x yields a pointer to an integer variable. The statement y := &x means that y points to x, or y holds the address of x. The expression *y retrieves the value stored in that integer variable, which in this case is 9.
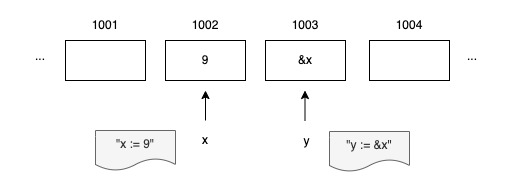

Chapter 2: The Importance of Pointers
Why are pointers advantageous? They enhance efficiency, as Go uses a pass-by-value approach for all data. Pointers allow you to pass the address of the data instead of its value. This prevents unintentional data alterations and enables you to access the actual value in another function rather than just a duplicate.
To illustrate this, consider the following examples:
Section 2.1: Pass by Value
When you pass by value, a copy of the variable's value is sent to the function. Any modifications made within the function only affect the function's local variable, leaving the original value unchanged.
Result:
User[Name: John, Age: 30]
User[Name: John, Age: 25]
Section 2.2: Pass by Pointer
In Go, since everything is passed by value, pointers are used to pass by reference and update the original value.
Result:
User[Name: John, Age: 30]
User[Name: John, Age: 30]
This video, "Golang Pointers - Fully Understanding Pointers in Go," provides further insights into the concept of pointers.
Additionally, the video "Go Pointers: When & How To Use Them Efficiently" discusses practical applications of pointers in Go programming.
If you found this article helpful, consider exploring my other writings on topics such as:
- How Do Golang Channels Work
- Observer Design Pattern in Golang with an Example
- Techniques for Retaining Classification Concepts Forever
Resources:
- Understand Go pointers in less than 800 words or your money back
- The Fundamentals of Pointers in Go