Mastering Continuous Profiling for Python Applications
Written on
Understanding Profiling
In the realm of computer programming, "profiling" refers to the process of pinpointing and optimizing resources that can lead to improved response times, reduced cache times, and minimized interruptions during the execution of a program. Essentially, profiling allows us to enhance a program’s execution speed while reducing CPU resource consumption. Given that most applications are now cloud-hosted, efficient resource utilization can lead to significant cost savings and enhanced code performance.
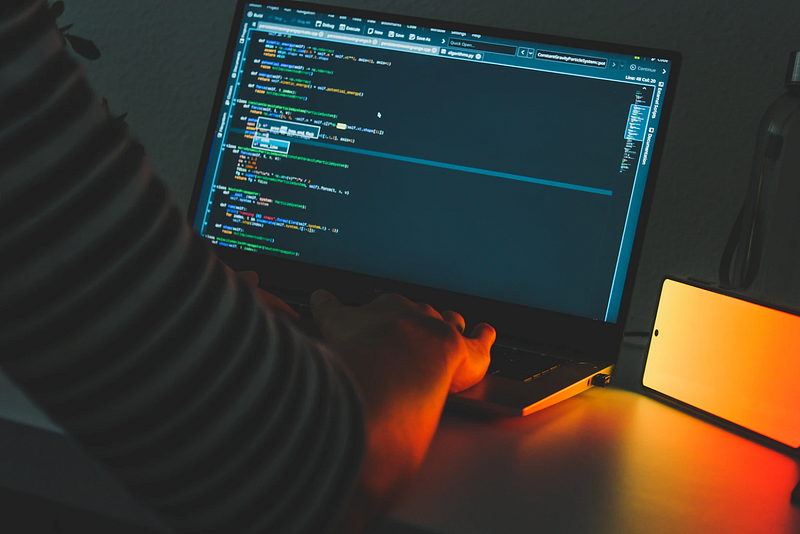
To implement profiling in any programming language, a profiler is necessary to gather various types of data aimed at accelerating execution and identifying bottlenecks, such as timing issues, function call execution, and caching problems. Profiling helps developers identify code segments that consume excessive execution time, allowing for optimization and better resource management. However, profilers should be employed judiciously, as they can slow down code execution and make it more complex to read. This is where gProfiler excels.
gProfiler simplifies the profiling process. It offers seamless production profiling without necessitating any changes to the code—it's truly plug-and-play.
Types of Profiling
Profiling can be categorized into two main types: deterministic and statistical profiling.
Deterministic Profiling
Deterministic profiling operates by executing a "trace" function at various points in the code, as specified by the developer, such as at function calls, returns, or exception occurrences. It helps to determine where a program is excessively consuming time or resources. While it can provide valuable insights into processing times, the calculations involved can also be resource-intensive. gProfiler mitigates this issue by functioning quietly in the background, using minimal resources.
Statistical Profiling
Statistical profiling focuses on the CPU state, assessing which procedures take too long to execute. This is managed by the CPU, which samples execution states at intervals. By analyzing these samples, developers can make informed decisions. gProfiler facilitates this by continually sampling at regular intervals, allowing for an ongoing evaluation of performance.
Video Description: In this video, Ryan Perry introduces continuous profiling using Pyroscope, emphasizing its benefits for developers.
Profiling Techniques in Python
Python boasts several robust modules for profiling application performance. These tools help identify where applications spend excessive time and how to improve efficiency. Continuous profiling is vital for enhancing program performance and ensuring optimal resource allocation. Two key modules in Python for profiling are "cProfile" and "profile." Let's explore them further.
I’ll demonstrate a simple Python snippet that performs addition. First, we’ll run it without profiling, then with profiling to observe any differences.
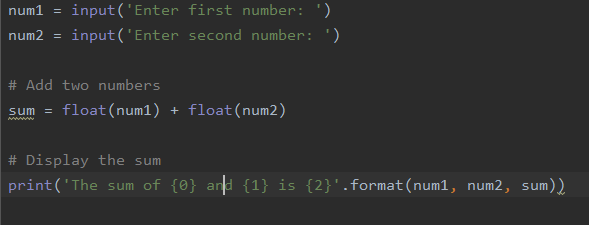
Normal Execution: Run this code using Python.

Execution with cProfile: To run with cProfile, we need to provide specific arguments, such as -m for module, using cProfile as our package.
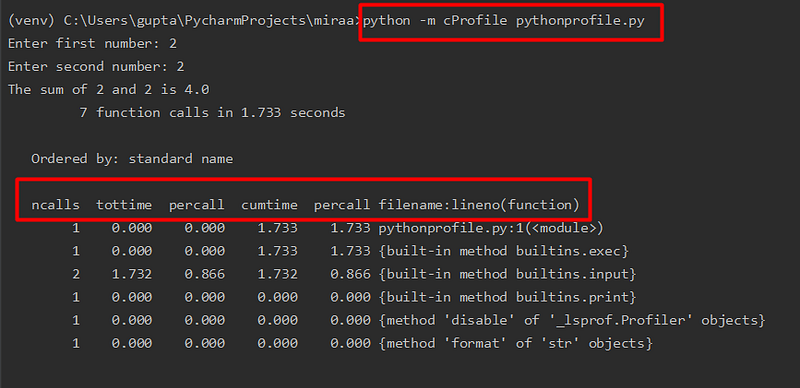
The difference in outputs reveals the execution time when utilizing cProfile. Key parameters include:
- ncalls: Number of calls made.
- totime: Total time spent executing the main operation, excluding sub-functions.
- cumtime: Cumulative time spent on both main and sub-functions.
- precall: Computes the ratio of totime to cumtime.
cProfile offers various options for sorting performance metrics according to our needs.

Targeted Profiling
Instead of profiling the entire code, targeted profiling allows us to focus on specific snippets. This approach is beneficial for analyzing performance without overwhelming the developer with data.
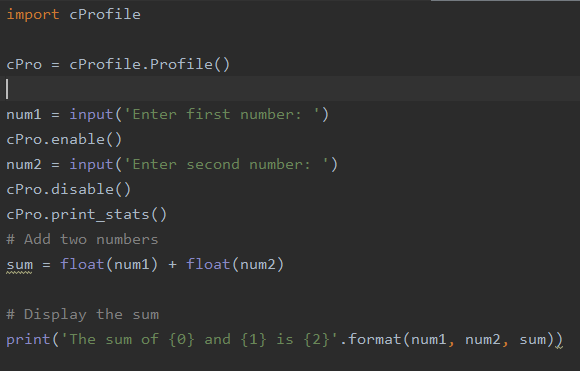
The output can vary significantly based on the size of the code snippet being analyzed.
Line Profiling
Line profiling examines the execution time of each line of code. This method is useful for optimizing performance by identifying which lines are most resource-intensive.
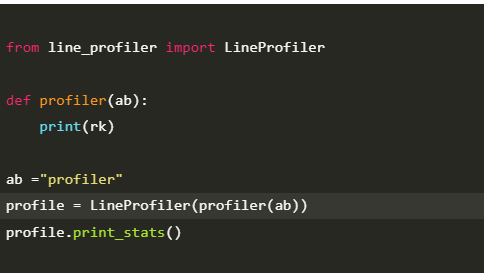
This technique requires additional dependencies that can be installed via pip. The profiling quality remains consistent, but the approach focuses on a granular level of detail.
Using gProfiler for Efficient Profiling
For profiling in Python, you typically need to install modules like cProfile or memory_profile. This can complicate the coding process. gProfiler resolves this issue by running in the background, allowing you to monitor code performance without modifying your codebase.
To install gProfiler on Ubuntu, follow these steps:
- Open the command line and provide your service name. You can use the same tool across multiple instances to monitor them from a single dashboard.
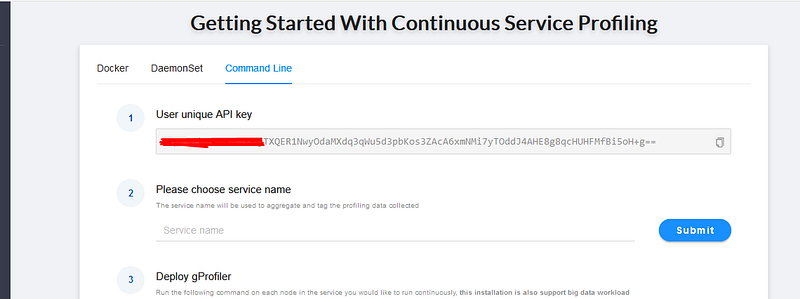
- Use the wget command provided on your dashboard to download gProfiler.
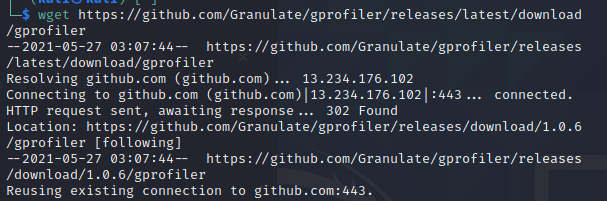
- After downloading, install and execute it to begin monitoring.
- Adjust permissions to allow execution.
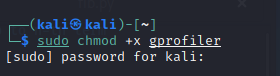
- Start the service.
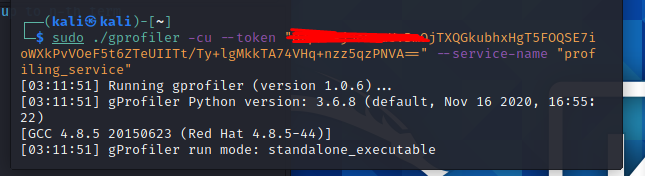
gProfiler will now monitor your Python applications, collecting statistics which are accessible via its dashboard. This tool operates in the background with minimal memory usage, providing you with valuable insights without requiring code modifications.
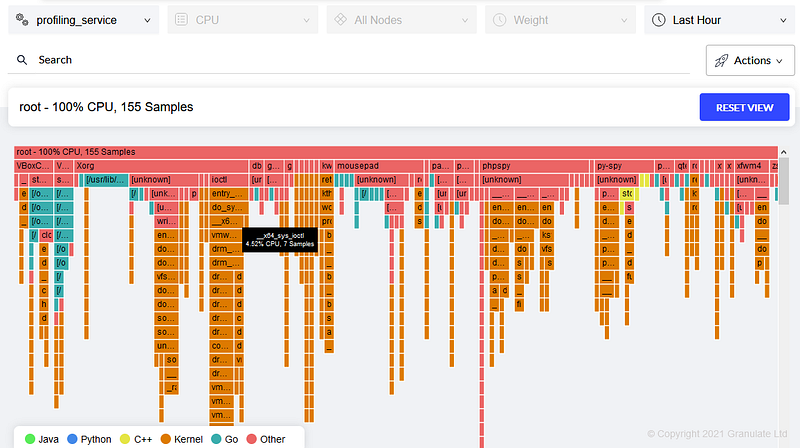
Conclusion
The principles discussed here are essential for effective profiling of Python programs. Profiling not only enhances code performance but also reduces CPU usage, leading to cost savings. Efficient resource management is crucial in today’s programming landscape.
Level Up Coding
Thank you for being a part of our community! Don’t forget to subscribe to our YouTube channel or join the Skilled.dev coding interview course.
Coding Interview Questions + Land Your Dev Job | Skilled.dev
The course to master the coding interview