Implementing a Retry Mechanism in Rust for Robust Error Handling
Written on
Chapter 1: The Importance of Error Handling
In software development, effective error handling is essential. Modern applications frequently communicate with external services via the internet, which can lead to temporary failures. One effective approach to increase resilience is by employing a retry mechanism. This article demonstrates how to seamlessly integrate retries into Rust functions, thereby improving your code's ability to manage failures gracefully.
Section 1.1: Adding Necessary Dependencies
Before we delve into the error-handling and retry implementation, it's important to ensure that we have the required dependencies. For our example, which involves making HTTP requests, we will use the reqwest crate. This library provides a user-friendly way to perform HTTP requests in Rust.
To include reqwest in your project, update your Cargo.toml file as follows:
[dependencies]
reqwest = { version = "0.11", features = ["blocking"] }
In this configuration, we specify version "0.11" of the crate and enable the "blocking" feature, which provides a synchronous interface suitable for simpler applications or scenarios where asynchronous execution is not required.
With this setup, you are now prepared to implement the retry logic in your Rust projects and effectively handle transient failures.
Section 1.2: Introducing the Retrier Struct
The foundation of our retry mechanism is the Retrier struct. This struct serves as a wrapper around an action (i.e., a function) that we want to execute, encapsulating the logic needed to retry the action multiple times with a defined delay between attempts.
Here’s a closer look at the struct:
struct Retrier<F> {
action: F,
max_retries: u32,
delay: std::time::Duration,
}
- action: The function we wish to execute and retry upon failure.
- max_retries: The maximum number of retry attempts.
- delay: The time to wait between each retry.
Section 1.3: Building the Retry Logic
We will implement the Retrier struct, allowing users to set the maximum number of retries and the delay duration. The Retrier struct includes builder-like methods (new, max_retries, and delay) for configuration, along with an execute method to run the action with retries.
The core functionality resides in the execute method, which calls the action and retries it when necessary. Each failed attempt results in an error message and a pause for the specified duration before trying again:
impl<F> Retrier<F>
where
F: Fn() -> Result<(), Box<dyn std::error::Error>>,
{
fn new(action: F) -> Self {
Self {
action,
max_retries: 5, // default
delay: std::time::Duration::from_secs(2), // default
}
}
fn max_retries(mut self, max_retries: u32) -> Self {
self.max_retries = max_retries;
self
}
fn delay(mut self, delay: std::time::Duration) -> Self {
self.delay = delay;
self
}
fn execute(&self) -> Result<(), Box<dyn std::error::Error>> {
for attempt in 1..=self.max_retries {
match (self.action)() {
Ok(_) => return Ok(()),
Err(e) => {
eprintln!(
"Attempt {} failed: {}. Retrying in {:?}",
attempt, e, self.delay
);
std::thread::sleep(self.delay);
}
}
}
Err("Maximum retries exceeded".into())
}
}
This implementation harnesses Rust's type system, ensuring that the action must be a function returning a Result with an error type compatible with Box<dyn Error>.
Chapter 2: A Practical Example: Fetching Data from a Web Service
Let’s explore a real-world example: retrieving data from a web service. Network requests can fail intermittently, making them perfect candidates for our retry mechanism:
fn fetch_web_service_data() -> Result<(), Box<dyn Error>> {
let response = reqwest::blocking::get(url)?;
if response.status().is_success() {
let content: String = response.text()?;
println!("Received data: {}", content);
Ok(())
} else {
Err(format!("Failed to fetch data. HTTP Status: {}", response.status()).into())}
}
This function attempts to retrieve data from a specified web service. If successful, it outputs the retrieved data. If it fails, it returns an error, which can be managed by our retry mechanism.
Gluing It All Together
Our main function illustrates how to utilize the Retrier:
use reqwest;
use std::error::Error;
fn main() {
let result = Retrier::new(fetch_web_service_data)
.max_retries(5)
.delay(std::time::Duration::from_secs(2))
.execute();
match result {
Ok(_) => println!("Successfully fetched data!"),
Err(e) => eprintln!("Failed to fetch data after retries: {}", e),
}
}
Here, we wrap the fetch_web_service_data function in a Retrier, configure the retry parameters, and execute it. If the action continues to fail, an error message will be displayed.
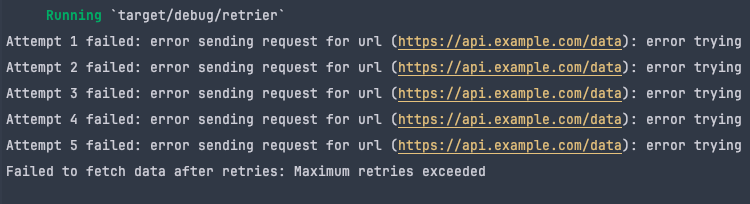
Conclusion
Rust, with its expressive syntax and robust type system, provides an excellent foundation for developing resilient and error-tolerant applications. The Retrier struct introduced here allows for an easy and flexible implementation of retry logic in any function. Whether you are fetching data from an external service, writing to a database, or handling other error-prone tasks, this retry mechanism can significantly enhance the reliability and robustness of your application.